Model Serializer
Automatically translate Odoo models into Datamodels for (de)serialization
Model Serializer
This module takes advantage of the concepts introduced in the datamodel module to offer mechanisms similar to (a subset of) the ModelSerializer in Django REST Framework. That is, use the definition of the Odoo model to partially automate the definition of a corresponding Datamodel class.
Important
This is an alpha version, the data model and design can change at any time without warning. Only for development or testing purpose, do not use in production. More details on development status
Table of contents
Usage
ModelSerializer
class
The ModelSerializer
class inherits from the Datamodel
class and adds functionalities. Therefore any
class inheriting from ModelSerializer
can be used the exact same way as any other Datamodel
.
Basic usage
Here is a basic example:
from odoo.addons.model_serializer.core import ModelSerializer class PartnerInfo(ModelSerializer): _name = "partner.info" _model = "res.partner" _model_fields = ["id", "name", "country_id"]
The result is equivalent to the following Datamodel
classes:
from marshmallow import fields from odoo.addons.datamodel.core import Datamodel from odoo.addons.datamodel.fields import NestedModel class PartnerInfo(Datamodel): _name = "partner.info" id = fields.Integer(required=True, allow_none=False, dump_only=True) name = fields.String(required=True, allow_none=False) country = NestedModel("_auto_nested_serializer.res.country") class _AutoNestedSerializerResCountry(Datamodel): _name = "_auto_nested_serializer.res.country" id = fields.Integer(required=True, allow_none=False, dump_only=True) display_name = fields.String(dump_only=True)
Overriding fields definition
It is possible to override the default definition of fields as such:
from odoo.addons.model_serializer.core import ModelSerializer class PartnerInfo(ModelSerializer): _name = "partner.info" _model = "res.partner" _model_fields = ["id", "name", "country_id"] country_id = NestedModel("country.info") class CountryInfo(ModelSerializer): _name = "country.info" _model = "res.country" _model_fields = ["code", "name"]
In this example, we override a NestedModel
but it works the same for any other field type.
(De)serialization
ModelSerializer
does all the heavy-lifting of transforming a Datamodel
instance into the corresponding
recordset
, and vice-versa.
To transform a recordset into a (list of) ModelSerializer
instance(s) (serialization), do the following:
partner_info = self.env.datamodels["partner.info"].from_recordset(partner)
This will return a single instance; if your recordset contains more than one record, you can get a list of instances
by passing many=True
to this method.
To transform a ModelSerializer
instance into a recordset (de-serialization), do the following:
partner = partner_info.to_recordset()
Unless an existing partner can be found (see below), this method creates a new record in the database. You can avoid
that by passing create=False
, in which case the system will only create them in memory (NewId
recordset).
In order to determine if the corresponding Odoo record already exists or if a new one should be created, the system
checks by default if the id
field of the instance corresponds to a database record. This default behavior can be
modified like so:
class CountryInfo(ModelSerializer): _name = "country.info" _model = "res.country" _model_fields = ["code", "name"] def get_odoo_record(self): if self.code: return self.env[self._model].search([("code", "=", self.code)]) return super().get_odoo_record()
Changelog
13.0.1.0.0
First official version.
Bug Tracker
Bugs are tracked on GitHub Issues. In case of trouble, please check there if your issue has already been reported. If you spotted it first, help us to smash it by providing a detailed and welcomed feedback.
Do not contact contributors directly about support or help with technical issues.
Credits
Authors
- Wakari
Contributors
- François Degrave <f.degrave@wakari.be>
Maintainers
This module is maintained by the OCA.
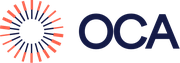
OCA, or the Odoo Community Association, is a nonprofit organization whose mission is to support the collaborative development of Odoo features and promote its widespread use.
This module is part of the OCA/rest-framework project on GitHub.
You are welcome to contribute. To learn how please visit https://odoo-community.org/page/Contribute.
Once the user has seen at least one product this snippet will be visible.