Base Rest
Develop your own high level REST APIs for Odoo thanks to this addon.
Base Rest
This addon provides the basis to develop high level REST APIs for Odoo.
As Odoo becomes one of the central pieces of enterprise IT systems, it often becomes necessary to set up specialized service interfaces, so existing systems can interact with Odoo.
While the XML-RPC interface of Odoo comes handy in such situations, it requires a deep understanding of Odoo’s internal data model. When used extensively, it creates a strong coupling between Odoo internals and client systems, therefore increasing maintenance costs.
Table of contents
Configuration
If an error occurs when calling a method of a service (ie missing parameter, ..) the system returns only a general description of the problem without details. This is done on purpose to ensure maximum opacity on implementation details and therefore lower security issue.
This restriction can be problematic when the services are accessed by an external system in development. To know the details of an error it is indeed necessary to have access to the log of the server. It is not always possible to provide this kind of access. That's why you can configure the server to run these services in development mode.
To run the REST API in development mode you must add a new section '[base_rest]' with the option 'dev_mode=True' in the server config file.
[base_rest] dev_mode=True
When the REST API runs in development mode, the original description and a stack trace is returned in case of error. Be careful to not use this mode in production.
Usage
To add your own REST service you must provides at least 2 classes.
- A Component providing the business logic of your service,
- A Controller to register your service.
The business logic of your service must be implemented into a component (odoo.addons.component.core.Component) that inherit from 'base.rest.service'
from odoo.addons.component.core import Component class PingService(Component): _inherit = 'base.rest.service' _name = 'ping.service' _usage = 'ping' _collection = 'my_module.services' # The following method are 'public' and can be called from the controller. def get(self, _id, message): return { 'response': 'Get called with message ' + message} def search(self, message): return { 'response': 'Search called search with message ' + message} def update(self, _id, message): return {'response': 'PUT called with message ' + message} # pylint:disable=method-required-super def create(self, **params): return {'response': 'POST called with message ' + params['message']} def delete(self, _id): return {'response': 'DELETE called with id %s ' % _id} # Validator def _validator_search(self): return {'message': {'type': 'string'}} # Validator def _validator_get(self): # no parameters by default return {} def _validator_update(self): return {'message': {'type': 'string'}} def _validator_create(self): return {'message': {'type': 'string'}}
Once your have implemented your services (ping, ...), you must tell to Odoo how to access to these services. This process is done by implementing a controller that inherits from odoo.addons.base_rest.controllers.main.RestController
from odoo.addons.base_rest.controllers import main class MyRestController(main.RestController): _root_path = '/my_services_api/' _collection_name = my_module.services
In your controller, _'root_path' is used to specify the root of the path to access to your services and '_collection_name' is the name of the collection providing the business logic for the requested service/
By inheriting from RestController the following routes will be registered to access to your services
@route([ ROOT_PATH + '<string:_service_name>', ROOT_PATH + '<string:_service_name>/search', ROOT_PATH + '<string:_service_name>/<int:_id>', ROOT_PATH + '<string:_service_name>/<int:_id>/get' ], methods=['GET'], auth="user", csrf=False) def get(self, _service_name, _id=None, **params): method_name = 'get' if _id else 'search' return self._process_method(_service_name, method_name, _id, params) @route([ ROOT_PATH + '<string:_service_name>', ROOT_PATH + '<string:_service_name>/<string:method_name>', ROOT_PATH + '<string:_service_name>/<int:_id>', ROOT_PATH + '<string:_service_name>/<int:_id>/<string:method_name>' ], methods=['POST'], auth="user", csrf=False) def modify(self, _service_name, _id=None, method_name=None, **params): if not method_name: method_name = 'update' if _id else 'create' if method_name == 'get': _logger.error("HTTP POST with method name 'get' is not allowed. " "(service name: %s)", _service_name) raise BadRequest() return self._process_method(_service_name, method_name, _id, params) @route([ ROOT_PATH + '<string:_service_name>/<int:_id>', ], methods=['PUT'], auth="user", csrf=False) def update(self, _service_name, _id, **params): return self._process_method(_service_name, 'update', _id, params) @route([ ROOT_PATH + '<string:_service_name>/<int:_id>', ], methods=['DELETE'], auth="user", csrf=False) def delete(self, _service_name, _id): return self._process_method(_service_name, 'delete', _id)
The HTTP GET 'http://my_odoo/my_services_api/ping' will be dispatched to the method PingService.search
Known issues / Roadmap
The roadmap and known issues can be found on GitHub.
Changelog
10.0.2.0.1
- _validator_...() methods can now return a cerberus Validator object instead of a schema dictionnary, for additional flexibility (e.g. allowing validator options such as allow_unknown).
10.0.2.0.0
- Licence changed from AGPL-3 to LGPL-3
10.0.1.0.1
- Fix issue when rendering the jsonapi documentation if no documentation is provided on a method part of the REST api.
10.0.1.0.0
First official version. The addon has been incubated into the Shopinvader repository from Akretion. For more information you need to look at the git log.
Bug Tracker
Bugs are tracked on GitHub Issues. In case of trouble, please check there if your issue has already been reported. If you spotted it first, help us smashing it by providing a detailed and welcomed feedback.
Do not contact contributors directly about support or help with technical issues.
Credits
Authors
- ACSONE SA/NV
Contributors
- Laurent Mignon <laurent.mignon@acsone.eu>
- Sébastien Beau <sebastien.beau@akretion.com>
Maintainers
This module is maintained by the OCA.
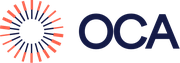
OCA, or the Odoo Community Association, is a nonprofit organization whose mission is to support the collaborative development of Odoo features and promote its widespread use.
Current maintainer:
This module is part of the OCA/rest-framework project on GitHub.
You are welcome to contribute. To learn how please visit https://odoo-community.org/page/Contribute.
Once the user has seen at least one product this snippet will be visible.